Next js, a popular React framework, introduces powerful features like Server-Side Rendering (SSR) and Static Site Generation (SSG) to enhance the performance and user experience of web applications. These techniques address the challenges of traditional client-side rendering and provide solutions for improved search engine optimization (SEO), faster loading times, and efficient data fetching.
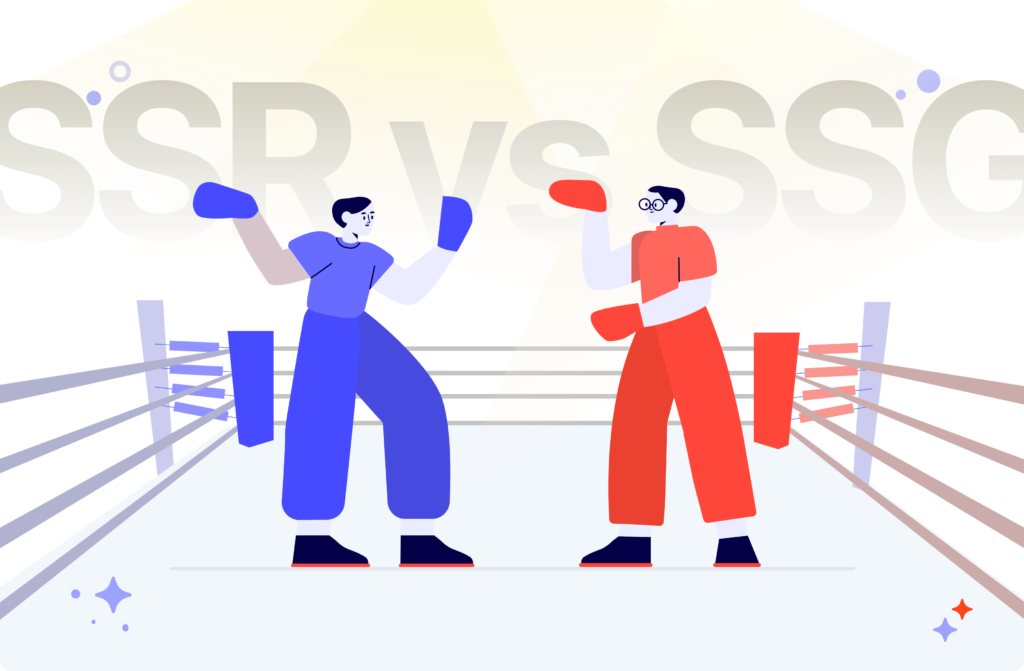
Table of Contents
Server-Side Rendering (SSR):
Server-Side Rendering is a technique where the server generates the HTML content for a page during runtime and sends it to the client, as opposed to the traditional approach where the client’s browser is responsible for rendering the entire page using JavaScript. SSR enhances performance by delivering pre-rendered content directly to the user, reducing the time it takes for a page to become interactive.
Next.js simplifies SSR by making it an integral part of its framework. To implement SSR in Next.js, you create a file in the pages
directory, and the framework automatically handles the server-side rendering. For example:
// pages/index.js
import React from 'react';
const HomePage = ({ data }) => (
<div>
<h1>{data.title}</h1>
<p>{data.content}</p>
</div>
);
export async function getServerSideProps() {
// Fetch data from an API or database
const res = await fetch('https://api.example.com/data');
const data = await res.json();
// Pass data as props to the component
return {
props: { data },
};
}
export default HomePage;
In above example, the getServerSideProps
function fetches data during the server-rendering process and passes it as props to the component. This ensures that the data is available when the page is initially loaded.
Static Site Generation (SSG):
Static Site Generation is a method where the HTML pages are generated at build time, and the pre-rendered content is served to users. This technique is particularly beneficial for content-heavy websites, blogs, or pages with relatively static data.
Next.js makes Static Site Generation straightforward by allowing developers to define which pages should be statically generated at build time. To implement SSG, you can use the getStaticProps
function:
// pages/blog/[slug].js
import React from 'react';
const BlogPost = ({ post }) => (
<div>
<h1>{post.title}</h1>
<p>{post.content}</p>
</div>
);
export async function getStaticPaths() {
// Fetch a list of paths (e.g., from an API or database)
const paths = await getPathsFromSomeSource();
// Specify paths to pre-render
return { paths, fallback: false };
}
export async function getStaticProps({ params }) {
// Fetch data for a specific post based on the slug
const post = await getPostBySlug(params.slug);
// Pass data as props to the component
return {
props: { post },
};
}
export default BlogPost;
In above example, getStaticPaths
generates a list of paths to pre-render based on available data, and getStaticProps
fetches the data for a specific post. The generated pages are stored as static files, making them easily distributable and cacheable.
Choosing Between SSR and SSG:
The choice between Server-Side Rendering and Static Site Generation depends on the nature of the application and its data requirements. SSR is suitable for pages with frequently changing data, where the most up-to-date content is crucial. SSG is ideal for content that doesn’t change often, providing a balance between performance and dynamic content.
Next js also offers a hybrid approach, allowing developers to combine SSR and SSG within the same application. This flexibility empowers developers to optimize different sections of a website based on specific needs.
In conclusion, Next js support for Server-Side Rendering and Static Site Generation offers developers versatile tools to enhance the performance, SEO, and user experience of their web applications. Whether choosing SSR, SSG, or a hybrid approach, Next.js provides an intuitive framework for building modern, efficient, and scalable web applications.
Use Server-Side Rendering (SSR) When:
- Dynamic Content Updates: If your web application requires frequently updated or real-time data, SSR is a good choice. Since SSR generates the content on each request, it ensures that users receive the most up-to-date information.
- Personalized Content: For pages with personalized content based on user authentication or specific user data, SSR is essential. It allows you to tailor the content dynamically for each user.
- Complex User Interfaces: If your application has highly interactive components that depend on server-side computations, SSR can improve the initial page load performance by rendering the HTML on the server.
- SEO Considerations: SSR is beneficial for SEO, as search engines can easily crawl and index the content rendered on the server. This is crucial for ensuring that your website ranks well in search engine results.
Use Static Site Generation (SSG) When:
- Static Content: SSG is ideal for websites with content that doesn’t change frequently. If the majority of your pages have relatively static data, SSG can significantly improve performance by generating and serving static files.
- Better Performance: Since SSG generates pages at build time, the resulting static files can be distributed globally on a Content Delivery Network (CDN), leading to faster loading times for users worldwide.
- Reduced Server Load: With SSG, your server is not involved in handling each request dynamically, reducing server load. This is particularly advantageous for websites with a high volume of traffic.
- Cost-Efficiency: Hosting static files on a CDN can be more cost-effective than managing server resources for dynamic content generation, especially for websites with a large user base.
Considerations for Both SSR and SSG:
- Hybrid Approach: Next.js, for example, allows for a hybrid approach where you can choose SSR for certain pages and SSG for others within the same application. This provides flexibility to optimize different sections based on specific requirements.
- Data Fetching: Both SSR and SSG support data fetching during the build process, but the choice between them may depend on how frequently the data changes. For dynamic data, SSR is more suitable, while SSG is ideal for relatively static data.
- User Experience: Consider the desired user experience. If immediate interactivity is crucial, SSR might be preferred for its ability to render content on each request. If faster initial page loads and overall performance are priorities, SSG may be the better choice.
Ultimately, the decision between SSR and SSG should align with your application’s goals, content dynamics, and user experience requirements. It’s also worth noting that the two approaches are not mutually exclusive, and a hybrid strategy might be the most suitable solution for certain applications.