A multi item carousel is a powerful component that can be used to showcase multiple items simultaneously in a sliding format. This is particularly useful for displaying products, services, testimonials, images, and other content that you want to present in an interactive, modern, and visually appealing way.
Bootstrap 5, the latest version of the popular CSS framework, provides an easy-to-implement carousel component that can be customized for various uses, including multi-item carousels. In this article, we’ll walk you through how to create a multi item carousel using Bootstrap 5, explain why you should use it, and provide examples of where it can be applied effectively.
Table of Contents
Why Use a Multi Item Carousel?
The multi-item carousel is a powerful UI feature for various reasons:
- Enhanced User Experience: Multi item carousels allow users to quickly browse through multiple items without leaving the page or scrolling endlessly. This keeps users engaged and improves their overall experience on your website.
- Efficient Space Utilization: Carousels are a great way to present multiple items in a limited space. Instead of listing products or testimonials in long rows, you can compact them into a slider, which makes your page look clean and organized.
- Interactive Design: Carousels add an interactive element to your website, making it more dynamic and appealing. Users can click through items at their own pace, creating a sense of control and engagement.
- Visually Appealing: Carousels are visually captivating and can effectively highlight important information such as featured products, services, or client testimonials. This can help increase conversions and attract attention to key areas of your site.
Where Can You Use a Multi Item Carousel?
Multi item carousels can be used in various places on your website, including:
- E-commerce Websites: Display multiple product images or product categories in a carousel format. For example, you could showcase bestsellers, new arrivals, or featured products all in one area.
- Portfolios: If you’re a designer, artist, or photographer, you can use a multi-item carousel to present a series of works or projects in a visually dynamic way.
- Client Testimonials: You can use a carousel to display multiple testimonials, giving users a quick way to browse through feedback from different clients.
- News or Blog Sites: Use a multi item carousel to display the latest articles, news updates, or featured stories in a sliding format.
- Marketing Campaigns: Carousels are great for showcasing multiple promotional banners, special offers, or ad campaigns.
Now, let’s dive into creating a multi-item carousel using Bootstrap 5.
Getting Started: Bootstrap 5 Setup
Before we begin creating our carousel, ensure you have Bootstrap 5 properly linked to your project. You can either download Bootstrap 5 or link to it via a CDN.
Here’s how you can include Bootstrap 5 via a CDN:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Multi-Item Carousel</title>
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha1/dist/css/bootstrap.min.css" rel="stylesheet">
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha1/dist/js/bootstrap.bundle.min.js"></script>
</head>
<body>
<!-- Your content here -->
</body>
</html>
Step 1: Basic Bootstrap 5 Carousel Setup
The first step is to create the basic structure of a Bootstrap carousel. Bootstrap 5 provides a carousel component that you can customize. Here’s a simple carousel with just one item per slide:
<div id="carouselExample" class="carousel slide" data-bs-ride="carousel">
<div class="carousel-inner">
<div class="carousel-item active">
<img src="image1.jpg" class="d-block w-100" alt="...">
</div>
<div class="carousel-item">
<img src="image2.jpg" class="d-block w-100" alt="...">
</div>
<div class="carousel-item">
<img src="image3.jpg" class="d-block w-100" alt="...">
</div>
</div>
<button class="carousel-control-prev" type="button" data-bs-target="#carouselExample" data-bs-slide="prev">
<span class="carousel-control-prev-icon" aria-hidden="true"></span>
<span class="visually-hidden">Previous</span>
</button>
<button class="carousel-control-next" type="button" data-bs-target="#carouselExample" data-bs-slide="next">
<span class="carousel-control-next-icon" aria-hidden="true"></span>
<span class="visually-hidden">Next</span>
</button>
</div>
In above setup:
- The carousel-inner class contains the slides of the carousel.
- Each carousel-item represents one slide. The first slide is marked as active.
The controls for previous and next slides are implemented using the carousel-control-prev and carousel-control-next classes.
Step 2: Modifying bootstrap 5 Carousel to Display Multiple Items
To convert this into a multi-item carousel, we’ll need to display multiple items within each slide. This can be done using Bootstrap 5 grid system.
Here’s how you can modify the carousel:
<div id="multiItemCarousel" class="carousel slide" data-bs-ride="carousel">
<div class="carousel-inner">
<div class="carousel-item active">
<div class="row">
<div class="col-md-4">
<img src="image1.jpg" class="d-block w-100" alt="...">
</div>
<div class="col-md-4">
<img src="image2.jpg" class="d-block w-100" alt="...">
</div>
<div class="col-md-4">
<img src="image3.jpg" class="d-block w-100" alt="...">
</div>
</div>
</div>
<div class="carousel-item">
<div class="row">
<div class="col-md-4">
<img src="image4.jpg" class="d-block w-100" alt="...">
</div>
<div class="col-md-4">
<img src="image5.jpg" class="d-block w-100" alt="...">
</div>
<div class="col-md-4">
<img src="image6.jpg" class="d-block w-100" alt="...">
</div>
</div>
</div>
</div>
<button class="carousel-control-prev" type="button" data-bs-target="#multiItemCarousel" data-bs-slide="prev">
<span class="carousel-control-prev-icon" aria-hidden="true"></span>
<span class="visually-hidden">Previous</span>
</button>
<button class="carousel-control-next" type="button" data-bs-target="#multiItemCarousel" data-bs-slide="next">
<span class="carousel-control-next-icon" aria-hidden="true"></span>
<span class="visually-hidden">Next</span>
</button>
</div>
In this updated code:
- We’re now using the grid system within each carousel-item. In this case, we have three items (col-md-4), which will be shown in each slide.
- You can adjust the number of items per slide by changing the grid column width (e.g., col-md-3 for four items per slide, col-md-6 for two items per slide).
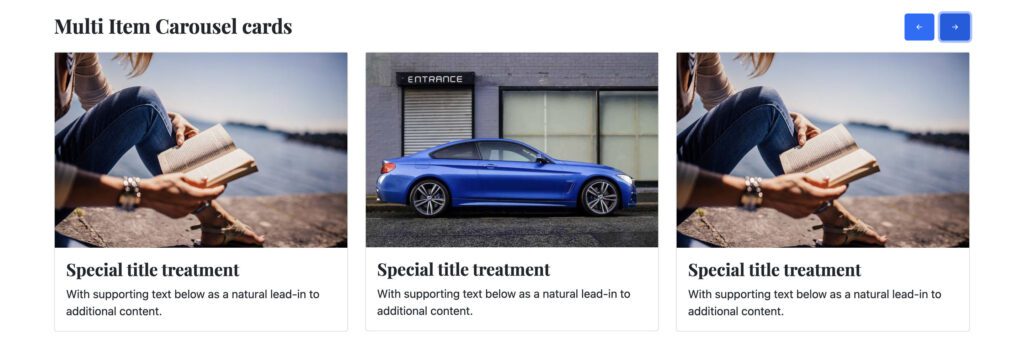
Step 3: Adding Responsiveness
To ensure the carousel is responsive, you can adjust the column sizes for different breakpoints. For example:
<div class="col-md-4 col-sm-6 col-xs-12">
<img src="image1.jpg" class="d-block w-100" alt="...">
</div>
This ensures that on smaller screens, the items will stack and display properly.
Step 4: Adding Custom Styles
You can further enhance your carousel with custom CSS. For example, you might want to add some spacing between the items:
.carousel-item img {
padding: 10px;
}
Or perhaps you want to add a background color to each slide:
.carousel-item {
background-color: #f8f9fa;
}
Step 5: Adding Captions
To make the carousel even more informative, you can add captions to each item:
<div class="col-md-4">
<img src="image1.jpg" class="d-block w-100" alt="...">
<div class="carousel-caption d-none d-md-block">
<h5>Item 1</h5>
<p>Short description of item 1.</p>
</div>
</div>
Where to Use Multi Item Carousels?
As mentioned earlier, multi-item carousels are versatile and can be used in various parts of a website. Here are some specific examples:
- E-commerce Homepages: Display your top products, promotional banners, or new arrivals using a multi item carousel to grab users’ attention.
- Portfolio Websites: Showcase multiple projects or artworks simultaneously in a clean, organized way.
- Blog or News Sites: Use
you can find more related blog to at my blog page